写了一个简单的串口测试工具,测试的是写入的速度。简单的说,就是打开串口,然后向里面写入数值,计算写入耗费的时间。通常来说,我们使用 USB 转串口设备,决定速度的因素有两个:1. USB 处理数据的时间 2.设备转串口的速度。其中最主要的因素是后者。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO.Ports; // For SerialPort
using System.Threading;
using System.IO;
using System.Diagnostics;
namespace _433CMD
{
class Program
{
const int COUNTER = 3;
static void Main(string[] args)
{
Stopwatch stopwatch = new Stopwatch();
if (args.Count() != 2)
{
Console.WriteLine("Please input COM PORT Number and ON or OFF");
Console.WriteLine("Usage:");
Console.WriteLine("SST COM3 115200");
Environment.Exit(1);
}
if (args[0].IndexOf("COM") == -1)
{
Console.WriteLine("Parameters error");
Environment.Exit(2);
}
int BaudRate;
if (int.TryParse(args[1], out BaudRate))
{
Console.WriteLine(BaudRate);
}
else
{
Console.WriteLine("BaudRate wrong");
}
SerialPort serialPort1 = new SerialPort();
serialPort1.PortName = args[0];
serialPort1.BaudRate = BaudRate;
serialPort1.DataBits = 8;
serialPort1.Parity = 0;
serialPort1.StopBits = (StopBits)1;
serialPort1.Encoding = System.Text.Encoding.GetEncoding(28591);
serialPort1.DtrEnable = true;
serialPort1.RtsEnable = true;
// Buffer 长1秒
Byte[] Buffer = new Byte[BaudRate/10];
stopwatch.Start();
try
{
// 打开串口
serialPort1.Open();
for (int i=0;i< COUNTER;i++)
{
serialPort1.Write(Buffer, 0, Buffer.Length);
}
serialPort1.Close();
}
catch (IOException e)
{
Console.WriteLine("Open " + args[1] + " failed ");
Console.WriteLine(e.Message);
Environment.Exit(4);
}
stopwatch.Stop();
TimeSpan ts = stopwatch.Elapsed;
Console.WriteLine("Send " + (Buffer.Length *COUNTER /1024).ToString() + "KB in " +(ts.TotalMilliseconds/1000).ToString("F3")+"s");
Console.WriteLine("Serial speed: "+(Buffer.Length * COUNTER/1024 / (ts.TotalMilliseconds/1000)).ToString("F3") +"KBytes/s");
Console.ReadLine();
}
}
}
使用 CH343 进行测试:
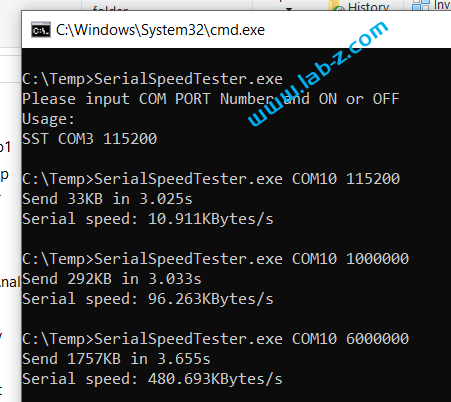
‘
编译后的 EXE 下载: