CRC-16 有很多种,这里使用的是CRC-16/CCITT-FALSE。可以用这个在线工具进行验证(注意下图是16进制数值):
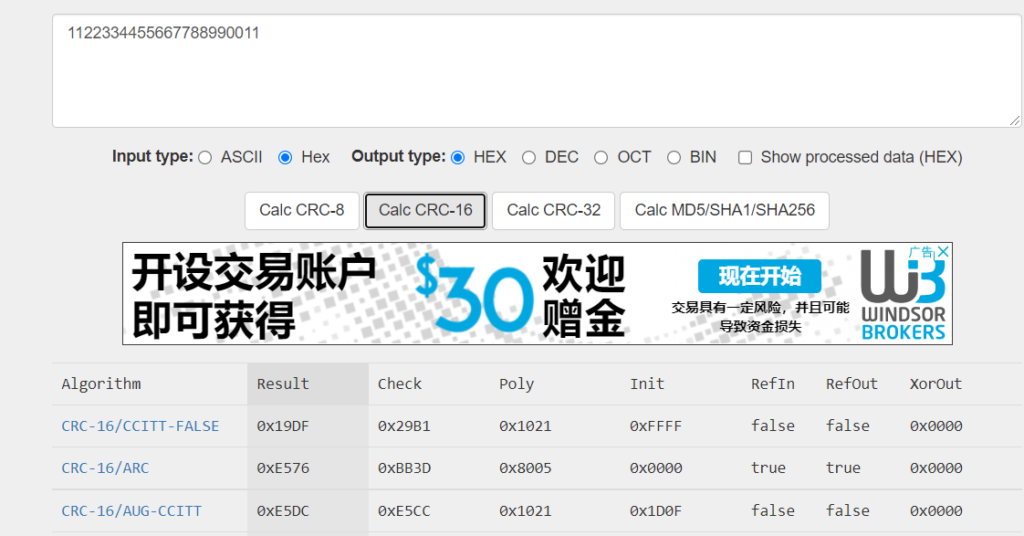
1.
static unsigned short crc16(const unsigned char *buf, unsigned long count)
{
unsigned short crc = 0xFFFF;
int i;
while(count--) {
crc = crc ^ *buf++ << 8;
for (i=0; i<8; i++) {
if (crc & 0x8000) crc = crc << 1 ^ 0x1021;
else crc = crc << 1;
}
}
return crc;
}
void setup() {
Serial.begin(115200);
}
void loop() {
byte data[11]={0x11,0x22,0x33,0x44,0x55,0x66,0x77,0x88,0x99,0x00,0x11};
Serial.println(crc16(data,11),HEX);
delay(10000);
}
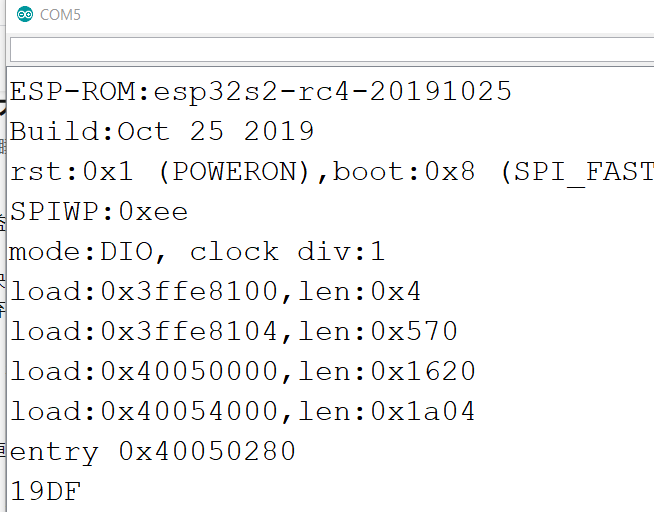
2.C# 代码
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Runtime.InteropServices;
namespace CRC16Test
{
class Program
{
static void Main(string[] args)
{
byte[] data = new byte[] { 0x11, 0x22, 0x33, 0x44, 0x55, 0x66, 0x77, 0x88, 0x99, 0x00,0x11};
/* for (int i = 0; i < 10; i++)
{
data[i] = (byte)(i % 256);
}
*/
string hex = Crc16Ccitt(data).ToString("x2");
Console.WriteLine(hex);
Console.Write("Press any key to continue . . . ");
Console.ReadKey(true);
}
//
// CRC-16/CCITT-FALSE
// https://crccalc.com/
//
public static ushort Crc16Ccitt(byte[] bytes)
{
const ushort poly = 0x1021;
ushort[] table = new ushort[256];
ushort initialValue = 0xffff;
ushort temp, a;
ushort crc = initialValue;
for (int i = 0; i < table.Length; ++i)
{
temp = 0;
a = (ushort)(i << 8);
for (int j = 0; j < 8; ++j)
{
if (((temp ^ a) & 0x8000) != 0)
temp = (ushort)((temp << 1) ^ poly);
else
temp <<= 1;
a <<= 1;
}
table[i] = temp;
}
for (int i = 0; i < bytes.Length; ++i)
{
crc = (ushort)((crc << 8) ^ table[((crc >> 8) ^ (0xff & bytes[i]))]);
}
return crc;
}
}
}
