Victor 86B 是一款带有 USB接口的数字万用表,就是说用户可以从USB口来获取当前的万用表测量结果。经过实验以及结合说明书,数字部分表示如下:
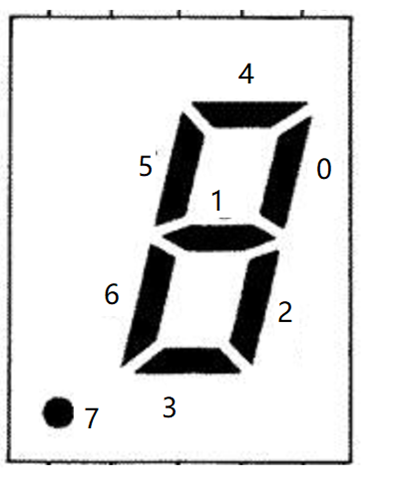
比如,“1” 可以通过 Bit0/2 置1来进行表示, 因此 “1”对应 0x05 (0000 0101B)。类似的,每个数字表示如下:
0 7D
1 05
2 5B
3 1F
4 27
5 3E
6 7E
7 15
8 7F
9 3F
有了这个表格,再配合说明编写一个 C# 的Windows Console程序:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Management;
using System.Diagnostics;
using System.Runtime.InteropServices;
// 程序中提到的接收 (read)和发送(write)都是以电脑端为主机的
namespace serialtest
{
public partial class Form1 : Form
{
public const int COM_BAUDRATE = 2400; //当前波特率
public Form1()
{
InitializeComponent();
}
byte[] readBuffer = new byte[1024]; //接收Buffer
private void Form1_Load(object sender, EventArgs e)
{
//设置可以选择的串口号
comboBox1.Items.AddRange(System.IO.Ports.SerialPort.GetPortNames());
comboBox1.SelectedIndex = 0;
//输出当前的串口设备名称
ManagementObjectSearcher searcher = new ManagementObjectSearcher("root\\CIMV2", "SELECT * FROM Win32_PnPEntity");
foreach (ManagementObject queryObj in searcher.Get())
{
if (queryObj["Caption"] != null)
if (queryObj["Caption"].ToString().Contains("(COM"))
{
textBox1.AppendText(queryObj["Caption"].ToString() + Environment.NewLine);
}
}
searcher.Dispose();
}
private void button1_Click(object sender, EventArgs e)
{
if (button1.Text == "PC Receive Stop")
{
// 开始接收 86B 发送过来的数据
serialPort1.BaudRate = COM_BAUDRATE;
serialPort1.PortName = comboBox1.SelectedItem.ToString();
// 为了接收> 127 的 ASCII 必须有下面这一句
serialPort1.Encoding = System.Text.Encoding.GetEncoding(28591);
// 设置收到 MAX_N 字节后触发
serialPort1.ReceivedBytesThreshold = 28;
serialPort1.Open();
button1.Text = "PC Receive Start";
}
else {
// 停止接收
button1.Text = "PC Receive Start";
serialPort1.Close();
}
}
private string ByteToString(byte b1,byte b2, Boolean first) {
byte tmp = (byte)(((byte)(b1 << 4)) + ((byte)(b2 & 0xF))&0x7F);
// 0 1 2 3 4 5 6 7 8 9 L
byte[] HexToValue = new byte[] { 0x7D, 0x05, 0x5B, 0x1F, 0x27, 0x3E, 0x7E, 0x15, 0x7F, 0x3F ,0x68};
String Result = "";
if (first) {
if ((b1 & 0x8) != 0) {
Result = Result + '-';
}
} else {
if ((b1 & 0x8) != 0)
{
Result = Result + '.';
}
}
for (int i = 0; i < 11; i++) {
if (tmp == HexToValue[i]) {
if (i == 10)
{ Result = Result + "L"; }
else { Result = Result + i.ToString(); }
break;
}
}
return Result;
}
private void serialPort1_DataReceived(object sender, System.IO.Ports.SerialDataReceivedEventArgs e)
{
// 当前处于接收模式
serialPort1.Read(readBuffer, 0, 28);
int start;
for (start = 0; start < 28; start++)
{
if ((byte)(readBuffer[start] & 0xF0) == 0x10) {
break;
}
}
// 收到的数据
textBox2.AppendText(">>>>>>>>>>>>>>>>\r\n");
for (int i = 0; i < 14; i++)
{
textBox2.AppendText(readBuffer[i+start].ToString("X2")+ " ");
}
textBox2.AppendText("\r\n");
if ((readBuffer[start] & 8) != 0) {
textBox2.AppendText("AC");
}
if ((readBuffer[start] & 4) != 0)
{
textBox2.AppendText("DC");
}
if ((readBuffer[start] & 2) != 0)
{
textBox2.AppendText("AUTO");
}
if ((readBuffer[start] & 1) != 0)
{
textBox2.AppendText("RS232\r\n");
}
textBox2.AppendText(
ByteToString(readBuffer[1+ start], readBuffer[2 + start],true)+
ByteToString(readBuffer[3 + start], readBuffer[4 + start],false)+
ByteToString(readBuffer[5 + start], readBuffer[6 + start], false) +
ByteToString(readBuffer[7 + start], readBuffer[8 + start], false)
);
//textBox2.AppendText("\r\n");
if ((readBuffer[9 + start] & 8) != 0) {
textBox2.AppendText("u");
}
if ((readBuffer[9 + start] & 4) != 0)
{
textBox2.AppendText("n");
}
if ((readBuffer[9 + start] & 2) != 0)
{
textBox2.AppendText("K");
}
if ((readBuffer[9 + start] & 1) != 0)
{
textBox2.AppendText("--->|---");
}
if ((readBuffer[10 + start] & 8) != 0)
{
textBox2.AppendText("m");
}
if ((readBuffer[10 + start] & 4) != 0)
{
textBox2.AppendText("% Duty");
}
if ((readBuffer[10 + start] & 2) != 0)
{
textBox2.AppendText("M");
}
if ((readBuffer[10 + start] & 1) != 0)
{
textBox2.AppendText("BEEP");
}
if ((readBuffer[11 + start] & 8) != 0)
{
textBox2.AppendText("F");
}
if ((readBuffer[11 + start] & 4) != 0)
{
textBox2.AppendText("Ω");
}
if ((readBuffer[11 + start] & 2) != 0)
{
textBox2.AppendText("REF");
}
if ((readBuffer[11 + start] & 1) != 0)
{
textBox2.AppendText("HOLD");
}
if ((readBuffer[12 + start] & 8) != 0)
{
textBox2.AppendText("A");
}
if ((readBuffer[12 + start] & 4) != 0)
{
textBox2.AppendText("V");
}
if ((readBuffer[12 + start] & 2) != 0)
{
textBox2.AppendText("Hz");
}
if ((readBuffer[12 + start] & 1) != 0)
{
textBox2.AppendText("BAT");
}
if ((readBuffer[13 + start] & 4) != 0)
{
textBox2.AppendText("mV");
}
if ((readBuffer[13 + start] & 2) != 0)
{
textBox2.AppendText("℃");
}
textBox2.AppendText("\r\n");
}
private void Form1_FormClosed(object sender, FormClosedEventArgs e)
{
serialPort1.Close();
}
}
}
运行结果:
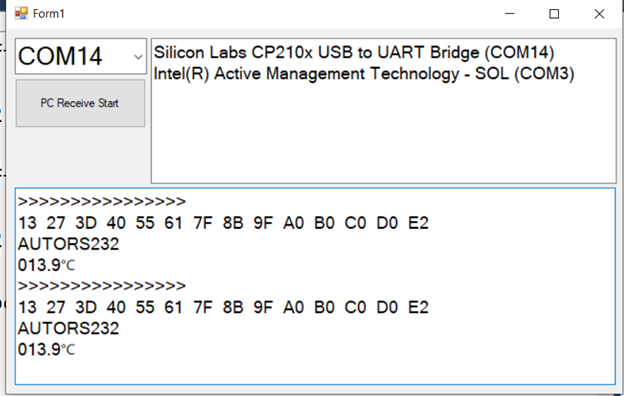