参考 http://embedded-lab.com/blog/tutorial-8-esp8266-internet-clock/ 这个代码,在 ILI9431 上实现模拟时钟的显示。
/***************************************************
This is our GFX example for the Adafruit ILI9341 Breakout and Shield
----> http://www.adafruit.com/products/1651
Check out the links above for our tutorials and wiring diagrams
These displays use SPI to communicate, 4 or 5 pins are required to
interface (RST is optional)
Adafruit invests time and resources providing this open source code,
please support Adafruit and open-source hardware by purchasing
products from Adafruit!
Written by Limor Fried/Ladyada for Adafruit Industries.
MIT license, all text above must be included in any redistribution
****************************************************/
#include "SPI.h"
#include "Adafruit_GFX.h"
#include "Adafruit_ILI9341.h"
// For the Adafruit shield, these are the default.
//#define ILI9341_DC 9
//#define ILI9341_CS 10
// For the Adafruit shield, these are the default.
#define ILI9341_DC D9
#define ILI9341_CS D8
#define ILI9341_MOSI MOSI
#define ILI9341_CLK SCK
#define ILI9341_RST D2
#define ILI9341_MISO MISO
// Use hardware SPI (on Uno, #13, #12, #11) and the above for CS/DC
Adafruit_ILI9341 tft = Adafruit_ILI9341(ILI9341_CS, ILI9341_DC, ILI9341_RST);
float sx = 0, sy = 1, mx = 1, my = 0, hx = -1, hy = 0; // Saved H, M, S x & y multipliers
float sdeg=0, mdeg=0, hdeg=0;
uint16_t osx=120, osy=120, omx=120, omy=120, ohx=120, ohy=120; // Saved H, M, S x & y coords
uint16_t x0=0, x1=0, yy0=0, yy1=0;
uint8_t hh, mm, ss;
void setup() {
Serial.begin(9600);
Serial.println("ILI9341 Test!");
tft.begin(2000000UL);
tft.fillScreen(ILI9341_NAVY);
tft.setTextColor(ILI9341_WHITE, ILI9341_DARKCYAN); // Adding a background colour erases previous text automatically
// Draw clock face
tft.fillCircle(120, 120, 118, ILI9341_GREEN);
tft.fillCircle(120, 120, 110, ILI9341_BLACK);
// Draw 12 lines
for(int i = 0; i<360; i+= 30) {
sx = cos((i-90)*0.0174532925);
sy = sin((i-90)*0.0174532925);
x0 = sx*114+120;
yy0 = sy*114+120;
x1 = sx*100+120;
yy1 = sy*100+120;
tft.drawLine(x0, yy0, x1, yy1, ILI9341_GREEN);
}
// Draw 60 dots
for(int i = 0; i<360; i+= 6) {
sx = cos((i-90)*0.0174532925);
sy = sin((i-90)*0.0174532925);
x0 = sx*102+120;
yy0 = sy*102+120;
// Draw minute markers
tft.drawPixel(x0, yy0, ILI9341_WHITE);
// Draw main quadrant dots
if(i==0 || i==180) tft.fillCircle(x0, yy0, 2, ILI9341_WHITE);
if(i==90 || i==270) tft.fillCircle(x0, yy0, 2, ILI9341_WHITE);
}
tft.fillCircle(120, 121, 3, ILI9341_WHITE);
}
void loop(void) {
for (hh=0;hh<12;hh++)
for (mm=0;mm<60;mm++)
for (ss=0;ss<60;ss++)
{
// Pre-compute hand degrees, x & y coords for a fast screen update
sdeg = ss*6; // 0-59 -> 0-354
mdeg = mm*6+sdeg*0.01666667; // 0-59 -> 0-360 - includes seconds
hdeg = hh*30+mdeg*0.0833333; // 0-11 -> 0-360 - includes minutes and seconds
hx = cos((hdeg-90)*0.0174532925);
hy = sin((hdeg-90)*0.0174532925);
mx = cos((mdeg-90)*0.0174532925);
my = sin((mdeg-90)*0.0174532925);
sx = cos((sdeg-90)*0.0174532925);
sy = sin((sdeg-90)*0.0174532925);
if (ss==0) {
// Erase hour and minute hand positions every minute
tft.drawLine(ohx, ohy, 120, 121, ILI9341_BLACK);
ohx = hx*62+121;
ohy = hy*62+121;
tft.drawLine(omx, omy, 120, 121, ILI9341_BLACK);
omx = mx*84+120;
omy = my*84+121;
}
// Redraw new hand positions, hour and minute hands not erased here to avoid flicker
tft.drawLine(osx, osy, 120, 121, ILI9341_BLACK);
osx = sx*90+121;
osy = sy*90+121;
tft.drawLine(osx, osy, 120, 121, ILI9341_RED);
tft.drawLine(ohx, ohy, 120, 121, ILI9341_WHITE);
tft.drawLine(omx, omy, 120, 121, ILI9341_WHITE);
tft.drawLine(osx, osy, 120, 121, ILI9341_RED);
tft.fillCircle(120, 121, 3, ILI9341_RED);
tft.setCursor(25, 245);
if(hh <10) tft.print(0);
tft.print(hh);
tft.print(':');
if(mm <10) tft.print(0);
tft.print(mm);
tft.print(':');
if(ss <10) tft.print(0);
tft.print(ss);
tft.setCursor(65, 285);
// tft.print(tzone);
//tft.drawCentreString("Time flies",120,260,4);
// delay(1000);
}
}
运行结果:
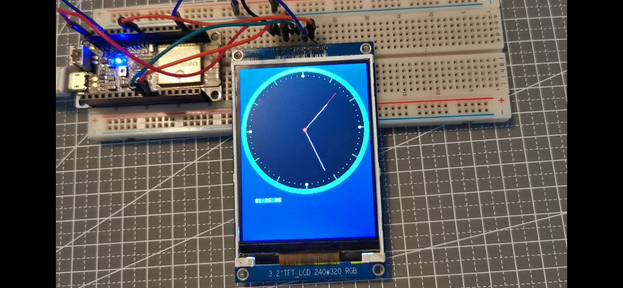
ILI9431 模拟时钟
文件下载: